Java Array: Sum of Elements in Even-Odd Positions
Arrays are fundamental data structures, and mastering their manipulation opens avenues for efficient programming. In this blog post, we’ll explore the Java programmatic approach to finding the sum of elements in even-odd positions within an array. Follow along for a comprehensive guide, practical coding examples, and insights into effective array traversal.
Understanding the Challenge:
Calculating the sum of elements in even and odd positions involves iterating through an array and selectively adding elements based on their index.
As we know that array index starts from Zero (0) means their 1st element position is the 0 and 5th element position is the 4 and so on.
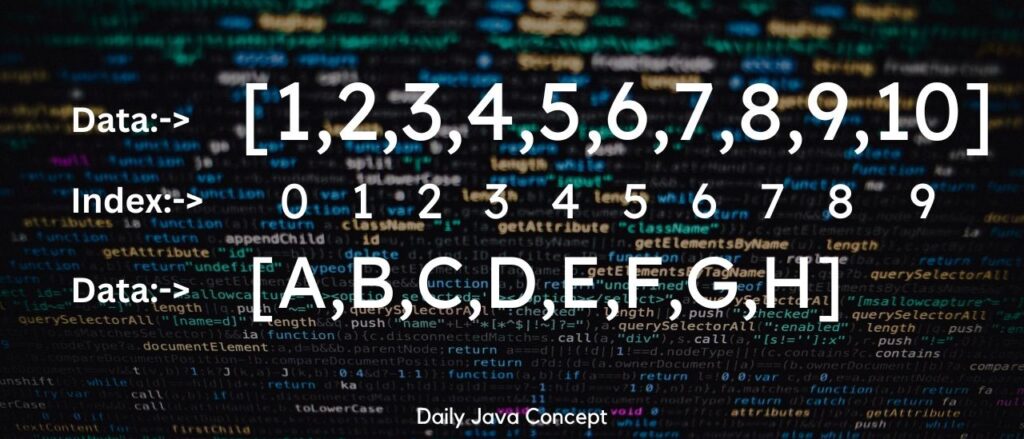
Before going to the program, you have to know that how to find even and odd numbers: Number is Even or Odd without modulus? after finding the position of the element based on odd/even you can sum the elements. Below program follows the modulus operator to find the even and odd numbers. this is also one of the learning.
Sum of Elements in Even-Odd Positions
public class EvenOddPositionSum {
public static void main(String[] args) {
// Declare and initialize an array
int[] array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Calculate sum of elements in even and odd positions
int evenPositionSum = 0;
int oddPositionSum = 0;
for (int i = 0; i < array.length; i++) {
if (i % 2 == 0) {
// Even position
evenPositionSum += array[i];
} else {
// Odd position
oddPositionSum += array[i];
}
}
// Display the results
System.out.println("Array: " + Arrays.toString(array));
System.out.println("Sum of elements in even positions: " + evenPositionSum);
System.out.println("Sum of elements in odd positions: " + oddPositionSum);
}
}
Output:
Array: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Sum of elements in even positions: 25
Sum of elements in odd positions: 30
Key Steps in Calculating Sum of Elements:
- Initialize the Array: Declare and initialize the array.
- Iterate Through the Array: Use a loop to traverse through each element.
- Check Even/Odd Position: Use the modulus operator to determine even or odd position.
- Calculate Sums: Accumulate the sum separately for even and odd positions.
- Display Results: Showcase the original array and the calculated sums.
Learn more about Array and their different use cases in Java: Array
Putting it All Together:
Finding the sum of elements in even and odd positions enhances your array manipulation skills. This knowledge becomes invaluable when dealing with data that follows a specific pattern.
Experiment with different arrays, observe the results, and gain confidence in navigating arrays in Java. This skill serves as a foundation for more complex data processing tasks. Happy coding!
Latest posts
- Merging Two Sorted Arrays in JavaTitle: Merging Two Sorted Arrays in Java Merging two sorted arrays into a single array is a common operation in… Read more: Merging Two Sorted Arrays in Java
- Frequency of Elements in an ArrayTitle: Frequency of Elements in an Array Using Java Counting the frequency of elements in an array is a fundamental… Read more: Frequency of Elements in an Array
- Calculate the sum of diagonals in a matrixTitle: Calculate the sum of diagonals in a matrix In this blog post, we’ll delve into the world of matrices… Read more: Calculate the sum of diagonals in a matrix
- Binary Search in JavaBinary search is a powerful algorithm used to efficiently find a target value in a sorted array. In this blog… Read more: Binary Search in Java
- Removing Duplicate Elements in Array using JavaRemoving Duplicate Elements in Array using Java Arrays are fundamental in Java, but duplicate elements can clutter your data. In… Read more: Removing Duplicate Elements in Array using Java
- Transpose a 2D Array in JavaTitle: Transpose a 2D Array in Java In Java, transposing a 2D array involves converting its rows into columns and… Read more: Transpose a 2D Array in Java