In the previous post, we learned about the Queue and their useful methods like Enqueue and Dequeue. It’s good for you to take some time and learn about the Queue if you don’t know about it. otherwise, continue the Queue Implementation in Java.
Queue Operation Implementation:
In Queue, there are the main two operations we can perform. Enqueue and Dequeue are respectively used for Inserting the Element in the Queue and deleting the Element from the Queue.
We are using the Queue related terminology in this post. It is good to understand topic related terminology before deep dive into the topic. Please find the below terminology useful:
Queue Related Term | General Meaning |
Enqueue | Inserting the element |
Dequeue | Deleting/removing the element |
To demonstrate the Queue Operation we are taking the 5 elements [10,20,30,40,50] to make you understand how Enqueue and Dequeue operation works in Queue.
Enqueue Operation:
Enqueue Operation is used to insert the new element into the Queue at the rear side.
In every element enqueued in Queue we simply assign New Node to the rear’s next Node and assign New Node as rear.
Step 1:
In the beginning the Queue is empty.
the pointer variables front and rear also pointing to the NULL in the beginning.
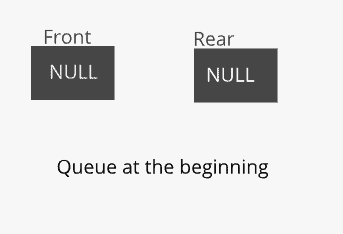
Step 2:
After enqueue the 1st element front and rear pointing the first element.
In Queue element enqueued at the rear side and deleted from the front side.
while enqueue the first element Condition Checked:
if (front == null){ front = rear = new_node; }
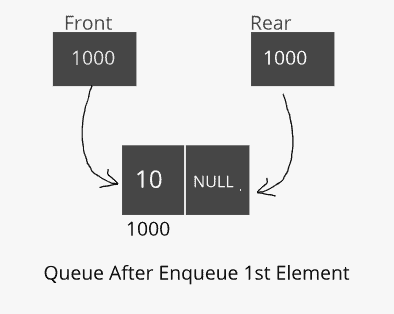
Step 3:
After enqueue the 1st element we are trying ti insert some more elements.
As we already said that, In Queue the element enqueued at the rear side. We are implementing the same in below code:
if{ rear.setNext(new_node); rear = new_node; }
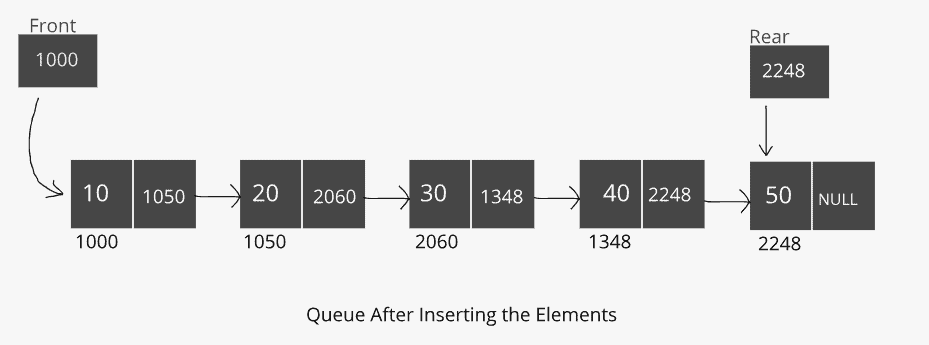
Dequeue Operation:
Dequeue operation is used to delete the element from the front side.
Each element dequeued from the Queue is simply assigning the front to next of front.
Step 1:
As we already said the element dequeued from the front side.
When we dequeue the element from the Queue. this time front pointing the 1st element of the Queue.
We can dequeue the element by simply assigning the front to front’s next Node.
We are implementing the same in the below code:
if (front.getNext() !=null){ front = front.getNext(); }
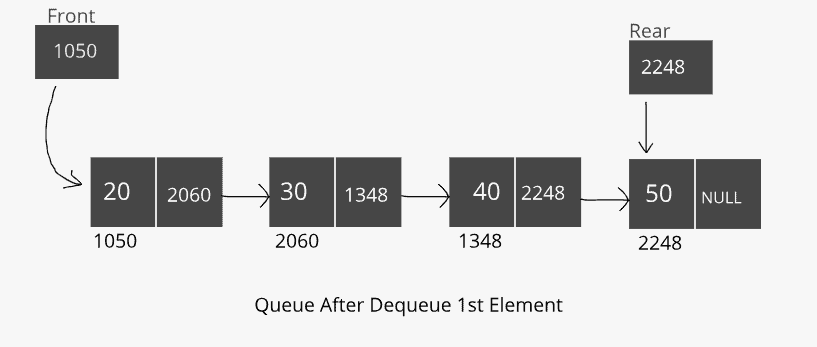
Step 2:
We are applying the same code to remove all the other elements from the queue except last element.
After dequeue all the element except last element both front and rear pointing the last element.
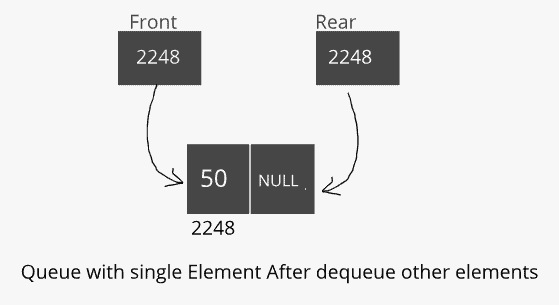
Step 3:
For dequeue the last element from the queue we simply set the front and rear both to null.
if{ front = null; rear = null; }
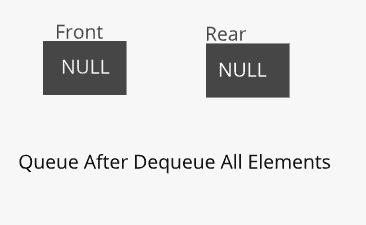
Queue Implementation in Java Code:
We are implementing the code in java divided into 3 parts to make you understand the Modularization and Code rec-usability.
The 1st Class called Node.java containing the Node implementation and useful methods to access and manipulate the Node easily.
The 2nd Class called Queue.java containing the Queue implementation and our own methods to access and manipulate the Queue easily.
The 3rd Class called QueueImplementation.java implements the Queue.java to perform some operation related to enqueue and dequeue.
Please find the Full code implementation below:
Node.java
public class Node { private int data; private Node next; public Node(int data) { this.setData(data); this.setNext(null); } public int getData() { return data; } public void setData(int data) { this.data = data; } public Node getNext() { return next; } public void setNext(Node next) { this.next = next; } }
Queue.java
public class Queue { private final int SIZE; public Node front; public Node rear; private int size = 0; public Queue(int SIZE) { front = null; rear = null; this.SIZE = SIZE; } public void enqueue(int ele){ Node new_node = new Node(ele); if (size == SIZE){ System.out.println("Queue Overflow\n Queue is Full."); }else{ if (front == null){ front = rear = new_node; }else{ rear.setNext(new_node); rear = new_node; } size++; } } public void dequeue(){ if (front !=null){ if (front.getNext() !=null){ front = front.getNext(); }else{ front = null; rear = null; } } } public void display(){ if (front == null) System.out.println("\nQueue is Empty!\n"); else { Node temp = front; while (temp != rear) { System.out.print(String.format("%d--->", temp.getData())); temp = temp.getNext(); } System.out.print(String.format("%d--->NULL", temp.getData())); } System.out.println(); } }
QueueImplementation.java
import java.util.Scanner; public class QueueImplementation { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Enter the size of the Queue: "); int n = sc.nextInt(); System.out.println("================================="); //Creating Queue Object with the User Defined Size // to access the implemented methods of Queue Queue queue = new Queue(n); System.out.println("Queue At the beginning : "); queue.display(); System.out.println("Front at Element : "+queue.front); System.out.println("Rear at Element : "+queue.rear); System.out.println("================================="); System.out.println("Enter the Elements : "); for (int i=0;i<n;i++){ int ele = sc.nextInt(); queue.enqueue(ele); } System.out.println("================================="); System.out.println("Queue After Enqueue the User Elements : "); queue.display(); System.out.println("Front at Element : "+queue.front.getData()); System.out.println("Rear at Element : "+queue.rear.getData()); queue.dequeue(); System.out.println("================================="); System.out.println("Queue After 1st Dequeue Operation : "); queue.display(); System.out.println("Front at Element : "+queue.front.getData()); System.out.println("Rear at Element : "+queue.rear.getData()); System.out.println("================================="); for (int i=0;i<n-1;i++){ queue.dequeue(); } System.out.println("Queue After Dequeue All Elements : "); queue.display(); System.out.println("Front at Element : "+queue.front); System.out.println("Rear at Element : "+queue.rear); System.out.println("================================="); } }
Output:
Enter the size of the Queue: 5 ================================= Queue At the beginning : Queue is Empty! Front at Element : null Rear at Element : null ================================= Enter the Elements : 10 20 30 40 50 ================================= Queue After Enqueue the User Elements : 10--->20--->30--->40--->50--->NULL Front at Element : 10 Rear at Element : 50 ================================= Queue After 1st Dequeue Operation : 20--->30--->40--->50--->NULL Front at Element : 20 Rear at Element : 50 ================================= Queue After Dequeue All Elements : Queue is Empty! Front at Element : null Rear at Element : null =================================
Hope you like this post.
If you have any doubt then feel free to comment below.
You will also need to learn below things related to Linked List: