In this post, we are going to learn a very important program that will asked in Interview very frequently. In Interview, It’s frequent that Interviewer will ask different methods for Java program to reverse a String. It may be in-build methods or without in-built methods like recursion.
Using StringBuffer and StringBuilder Class:
Both StringBuffer and StringBuilder class available in java.lang package and reverse() method is available in both classes. You can use any of the class to reverse a String. StringBuffer was introduced in Java 1.0 and StringBuilder was introduced in Java 1.5 version. This way you can say that StringBuilder is advanced version of StringBuffer.
In the below program we are implementing StringBuilder class to reverse a String:
import java.util.Scanner;
public class ReverseString {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the Input String: ");
String str = sc.next();
StringBuilder sb = new StringBuilder(str);
sb.reverse();
System.out.println("Reversed String : "+sb);
/*StringBuffer sb1 = new StringBuffer(str);
sb1.reverse();
System.out.println("Input String "+
str+" reversed Using StringBuffer: "+sb1);*/
}
}
Output:
//Output for the above program:
Enter the Input String: DailyJavaConcept
Reversed String : tpecnoCavaJyliaD
I commented out the StringBuffer implementation also, so if you want to use that you can code the same.
Iterative Method:
In the Iterative method we first convert a String array to Character array by using toCharArray() method. This method is available in String class. Character is the smallest part of any String so we will break it into characters then read the character from end to print the reverse of a String.
import java.util.Scanner; public class ReverseString { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the Input String: "); String str = sc.nextLine(); char[] stringArray = str.toCharArray(); for (int i = stringArray.length - 1;i>=0;i--){ System.out.print(stringArray[i]); } } }
Enter the Input String: DailyJavaConcept tpecnoCavaJyliaD
Enter the Input String: Hello from DailyJavaConcept tpecnoCavaJyliaD morf olleH
Explanation:
So what’s happening in the above code. Just come here and check this below:
First we are converting a String to Array of Character. For Example we have DailyJavaConcept in the Input. So in the starting this value stored in String. Then we converted this input string into Character Array: [‘D’,’a’,’i’,’l’,’y’,’J’,’a’,’v’,’a’,’C’,’o’,’n’,’c’,’e’,’p’,’t’].
Then by using for loop starting line number 12 we are reading this character array from the end means first character ‘t’ will be read and printed into console the, ‘p’ and so on till the character ‘D’. This way the values will be printed in reverse with the help of for loop.
Recursive method:
Recursion or Recursive Method means when a function/method call itself multiple time to find the Solution of the problem. Later, we can learn more about recursion.
By using recursion we write a code where method calls itself multiple times to reverse the String:
public String ReverseStringRecursive(String str){ if (str == null || str.length()<1){ return str; } return ReverseStringRecursive(str.substring(1))+str.charAt(0); }
Method written above is a Recursive method because If you see the Line Number 6 the method is calling itself again.
If the input string is null value then it will return the input string and if the Input value is not null then it will first take the first character of the string using str.charAt(0) apart and again call the ReverseStingRecursive() with the leftover string using the str.substring(1) method.
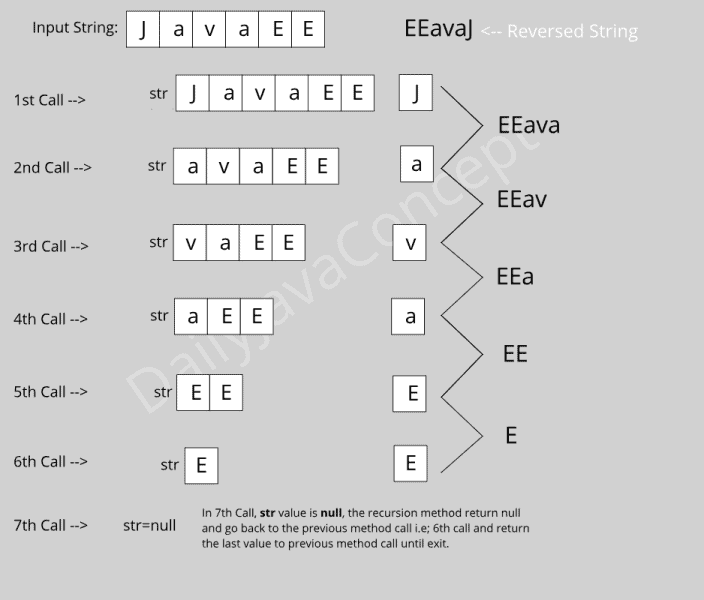
import java.util.Scanner; public class ReverseString { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the Input String: "); String str = sc.nextLine(); System.out.println("Reversed String is: "+ReverseStringRecursive(str)); } public static String ReverseStringRecursive(String str){ if (str == null || str.length()<1){ return str; } return ReverseStringRecursive(str.substring(1))+str.charAt(0); } }
Enter the Input String: DailyJavaConcept Reversed String is: tpecnoCavaJyliaD
If you have noticed that the Recursive method mention above and in the program itself has1 change. The above block of code has no static keyword in the method but in the program I added static in the method declaration.why?
Because In the program, I am calling ReverseStringRecursive() from the main method that is static method and every time you are accessing any method from main that should be static.
Hope you have liked this post about Java program to reverse a String.
Feel free to comment if you have any suggestion or need help.
feel free to learn more using below links:
- Arrays in Java
- Different ways to find the length of the array
- Java Pattern Programs
- How to run your Java Program?
- How to take user Input in Java?
- Data Structure in Java
- Linked List Implementation in Java