How to take user input in Java program?
To solve any problem we need to analyze the problem and after solving the problem and need the input to test the problem’s solution. In this post we are going to demonstrate you how to take user input in Java Program?
As in java or any programming language we mostly use command line to take the input. In this post I am going to demonstrate you how to take user input in Java program in 3 ways.
3 Ways to take input in Java using Command Line
Below are the 3 methods to take input in Java. you already know some of them but it is good to know all of them:
- Using String[] args
- Using Scanner Class
- Using IO Class
For using the Scanner Class and IO class we need to import the respective Classes in order to use them.
Using String[] args to take user input from Command Line in Java
In Java everything is String means the developer of Java given a more importance to String than any other Data type, but don’t think String is a Data type in Java. As String is a Class in Java so in order to use String we need to create the Object of String Class. Don’t confuse yourself, we will talk about String later.
String is very respected in Java so String array is given in the Syntax of main method to take user input in the form of String.
Java Program to demonstrate String[] args Method:
public class CommandLine { public static void main(String[] args) { String id = args[0]; String name = args[1]; String marks = args[2]; System.out.println("Id is : "+id); System.out.println("Name is : "+name); System.out.println("Marks is : "+marks); } }
You can directly use the args
array at the time of Printing thr values.
System.out.println(“Id is : “+args[0]);
System.out.println(“Name is : “+args[1]);
System.out.println(“Marks is : “+args[2]);
Save the above program with the name as Class name CommandLine.java
.
Execute the program using the CMD/Terminal.
first compile the program with javac
Command.
javac CommandLine.java
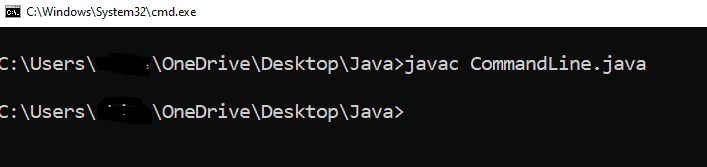
then run the program using the java
command with the input values. As in our program we need 3 input values so put the values as shown below:
java CommandLine 10 DailyJavaConcept 100
![Print the input values taken by String[] args method](https://dailyjavaconcept.com/wp-content/uploads/2020/07/image-1.png)
you have to put the values at the time of running the Java program separated by spaces.
Also always remember the sequence of the input values to use in the Program.
Using Scanner Class to take user input from Command Line in Java
Scanner class provides the Command line Input/Output feature in Java. But here we are only talking about the Input feature of Scanner Class. In order for using Scanner class we need to import the Scanner Class.
import java.util.Scanner;
using Scanner class we also need to create the Object of Scanner Class.
Scanner sc = new Scanner(System.in);
Scanner class provides multiple methods to take the input from the Command Line.
Java Program to demonstrate Scanner Class Method:
//Import Syntax of Scanner Class import java.util.Scanner; public class CommandLine { public static void main(String[] args) { //Object of Scanner Class Scanner sc = new Scanner(System.in); System.out.println("Enter your ID : "); int id = sc.nextInt(); System.out.println("Enter your Marks : "); float marks = sc.nextFloat(); System.out.println("Enter your Name : "); String name = sc.next(); System.out.println("Id is : "+id); System.out.println("Name is : "+name); System.out.println("Marks is : "+marks); } }
Scanner class provides the methods to take input of your desired type. like for Integer nextInt()
, for String next()
without Spaces, for Float values nextFloat()
and for Boolean type values nextBoolean()
. There are more methods but we will learn about it later.
In Scanner class Scanner Class hold the Output screen for the Human interaction to take the input.
When you run the program then you will be prompted to insert the values one by one as described in the program.
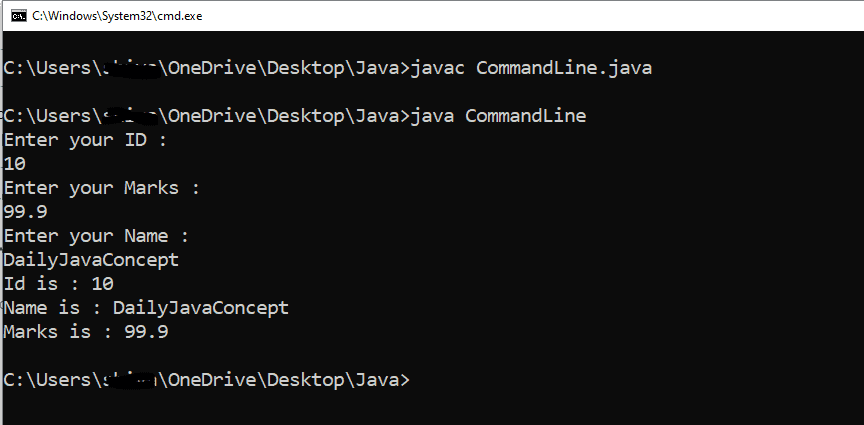
This is recommended to use to take the input from the CommandLine.
If you want to more about Scanner Class then Follow the Documentation link: Scanner Class
Using IO Class to take user input from Command Line in Java
The IO Class or Input-Output Class is specially written to take the input from different sources and write the output in different sources.
Using IO package we can read data from a file and also write output into the file.
As in this post we are talking about to take input from the command Line, so we only focus on those Classes and Methods that fulfil our requirement.
In order to use the IO Package classes we need to first import it on our Program.
import java.io.*;
In the last we are not specified any Class because we are using multiple classes to take the input from the command Line.
We need to create the Object of the InputStreamReader
that converts the Stream of Bytes into Characters.
InputStreamReader isr = new InputStreamReader(System.in);
Next we will create the object of the BufferedReader
that reads the text from the Characters that taken by the InputStreamReader
to provide the efficient reading.
BufferedReader br = new BufferedReader(isr);
Below program demonstrates you how to use IO Class to take input from the Commad Line.
Java Program to demonstrate Scanner Class Method:
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class CommandLine { public static void main(String[] args) throws IOException { //Object of InputStreamReader Class InputStreamReader isr = new InputStreamReader(System.in); //Object of BufferedReader Class BufferedReader br = new BufferedReader(isr); System.out.println("Enter your ID : "); //Convert String Input type to Integer and saved to corresponding Variable int id = Integer.parseInt(br.readLine()); //Convert to Float type and saved to corresponding Variable System.out.println("Enter your Marks : "); float marks = Float.parseFloat(br.readLine()); System.out.println("Enter your Name : "); String name = br.readLine(); System.out.println("Id is : "+id); System.out.println("Name is : "+name); System.out.println("Marks is : "+marks); } }
IO Package Classes may produces the Error at the runtime so it’s good to use try-catch block with IOException or simply throw the Exception on method level.
BufferedReader has a method to read String type values readLine()
. After taking the String type input, we will convert it to the desired datatype.
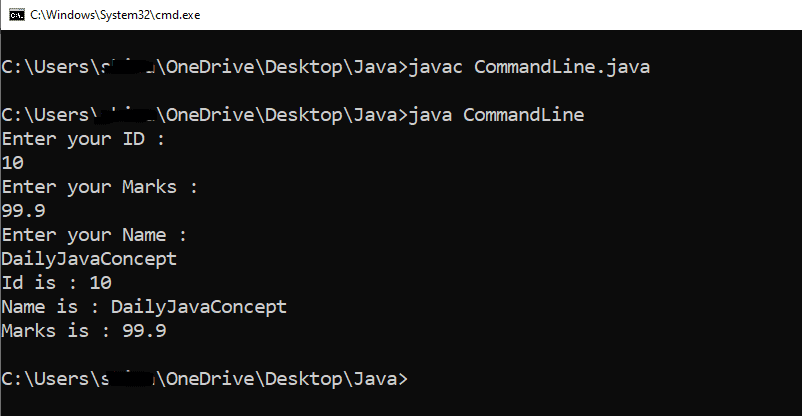
IO Class also wait for the input and prompted the corresponding message that is written in the program.
If you want to learn more then you follow the Documentation link: IO Package
Hope you enjoy this post and also learned a lot about How to take user input in Java program? in case you confused somewhere or you want to ask something then feel free to comment below.
You could find the below post useful for your knowledge:
- History of Java
- Java Programming Language
- Christmas Tree Pattern Program
- Data Structure in Java
- Queue implementation in Java
- Pattern Programs in Java
- Feature of Java
- Java Hello World Program
- How to install JDK in your Computer?